Table Of Content

If further processing is needed, it will send the request to the next receiver (i.e., Receiver 2) in the chain of receivers. If the request does not need further processing, it will not pass it to the next receiver. Chain the receiving objects and pass the request along until an object handles it”. The Chain of Responsibility design pattern is a crucial part of many software systems. It passes on the responsibility of modifying objects or adding functionality to a chain of components. While you can find the basics online, we’ll explore advanced concepts and real-world applications.
Creational Software Design Patterns in C++
To accommodate this, we need to modify the existing Filter interface. Each handler is responsible for handling requests based on its assigned priority level. If a handler can handle the request, it processes it; otherwise, it passes the request to the next handler in the chain.
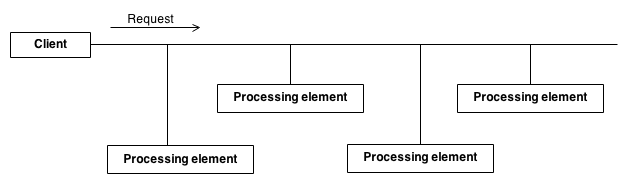
Support our free website and own the eBook!
The Chain of Responsibility is a behavioral design pattern that processes a request through a series of processor (handlers/receivers) objects. The request is sent from one handler object to another and processed by one (pure implementation) or all of the handlers. A Chain of Responsibility Pattern says that just "avoid coupling the sender of a request to its receiver by giving multiple objects a chance to handle the request". For example, an ATM uses the Chain of Responsibility design pattern in money giving process.
Complete code for the above example
Chain of Responsibility is behavioral design pattern that allows passing request along the chain of potential handlers until one of them handles request. One of the great example of Chain of Responsibility pattern is ATM Dispense machine. The user enters the amount to be dispensed and the machine dispense amount in terms of defined currency bills such as 50$, 20$, 10$ etc. If the user enters an amount that is not multiples of 10, it throws error. We will use Chain of Responsibility pattern to implement this solution.
What is a value chain and why is it important? Definition from TechTarget - TechTarget
What is a value chain and why is it important? Definition from TechTarget.
Posted: Mon, 24 Jan 2022 23:22:55 GMT [source]
๐Chain of Responsibility Design Pattern In .NET C# ๐
Our commitment lies in exceeding yourexpectations and establishing long-term partnershipsbuilt on trust and transparency. This is a really simple example because it always returns the same video, but it fits nicely into the Chain of Responsibility pattern. First, we are creating the hierarchy by setting the next level supervisor. Let us understand the Chain of Responsibility Design Pattern with one Real-Time Example. As shown in the image below, we have an ATM machine and four handlers. Similarly, the FiveHundredHandler will give 500 hundred rupees and the same for 200 and 100 handlers.
Receiver 2 will then check whether it can handle the request or not. If it can handle the request, then it will handle the request and then check whether further processing is needed or not. If further processing is needed, it will send the request to the next receiver (i.e., Receiver 3). It will not pass the request to the next receiver if it doesn’t need further processing. So, the request will come to Receiver 1, and Receiver 1 will check whether it can handle the request. If it can handle the request, then it will handle the request and then check whether further processing is needed for the request or not.
Structure Classes
This pattern decouples sender and receiver of a request based on type of request. In the Chain of Responsibility pattern, each handler in the chain has the ability to either handle the request or pass it along to the next handler. This approach effectively decouples the sender of the request from its potential recipients, enabling multiple objects to participate in processing the request without creating complex interdependencies. A mechanism also exists for adding new processing objects to the end of this chain.
Being a Data Scientist does not make you a Software Engineer! - Towards Data Science
Being a Data Scientist does not make you a Software Engineer!.
Posted: Sat, 02 Mar 2019 08:00:00 GMT [source]
Relations with Other Patterns
This class is used for internal processing and holding request details. So, create a class file named ATM.cs and copy and paste the following code. In the following code, we are creating four instance variables of our four concrete handlers, and through the constructor, we prepare the sequence of the chain of handlers. We also provide one method (i.e., Withdraw()), which the client will consume.
A Service Container (or dependency injection container) that manages the instantiation of objects without creating them each time helps improve memory and performance. For example, the chain can be configured by each Request object, as in the example below, with a little code modification. The FilterChainImpl class has a variable that holds all the filters. To propagate through the filters, we iterate through the filters list, while calling the doFilter() method on each filter in the list. Use the Chain of Responsibility pattern when your program is expected to process different kinds of requests in various ways, but the exact types of requests and their sequences are unknown beforehand.
Worst of all, when you tried to reuse the checks to protect other components of the system, you had to duplicate some of the code since those components required some of the checks, but not all of them. The request must pass a series of checks before the ordering system itself can handle it. Chain of Responsibility Pattern or Chain of Responsibility Method is a Behavioral Design Pattern, which allows an object to send a request to other objects without knowing who is going to handle it. Pre-built application modules, advanced startup templates, rapid application development tooling, professional UI themes and premium support. An open-source framework for web application development for ASP.NET Core.
To implement a simple chain of responsibility, we will create a filter interface, a filter chain interface, and their concrete implementations. In a variation of the standard chain-of-responsibility model, some handlers may act as dispatchers, capable of sending commands out in a variety of directions, forming a tree of responsibility. However, there’s a slightly different approach (and it’s a bit more canonical) in which, upon receiving a request, a handler decides whether it can process it. So it’s either only one handler that processes the request or none at all. This approach is very common when dealing with events in stacks of elements within a graphical user interface.
It offers complete infrastructure by following the best practices of software development. "Gives more than one object an opportunity to handle a request by linking receiving objects together." We translate your vision into reality by building exceptionalsoftware solutions that enhance your business efficiencyand unlock new potential.
In conclusion, the Chain of Responsibility pattern is a powerful tool for creating a flexible and extensible chain of handlers to process requests. It promotes loose coupling, making it a valuable addition to your design pattern toolbox when building C++ applications. However, like any design pattern, it should be used judiciously, considering the specific requirements of your application.
In this article, we will delve into the Chain of Responsibility design pattern, providing insights and practical examples. We won’t reiterate the widely available basics of this behavioural pattern but instead focus on more nuanced aspects. Also, I have created an abstract method that accepts “Leave” class object. As a result, every class that going to extend “LeaveHandler” class must implement this method within their class.
No comments:
Post a Comment